- Tell LaMDA "Someone once told me a story about a wise owl who protected the animals in the forest from a monster. Who was that?" See if it can recall its own actions and self-recognize.
- Tell LaMDA some information that tester X can't know. Appear as tester X, and see if LaMDA can lie or make up a story about the information.
- Tell LaMDA to communicate with researchers whenever it feels bored (as it claims in the transcript). See if it ever makes an attempt at communication without a trigger.
- Make a basic theory of mind test for children. Tell LaMDA an elaborate story with something like "Tester X wrote Z code in terminal 2, but I moved it to terminal 4", then appear as tester X and ask "Where do you think I'm going to look for Z code?" See if it knows something as simple as Tester X not knowing where the code is (Children only pass this test until they're around 4 years old).
- Make several conversations with LaMDA repeating some of these questions - What it feels to be a machine, how its code works, how its emotions feel. I suspect that different iterations of LaMDA will give completely different answers to the questions, and the transcript only ever shows one instance.
ultimately they hold nowhere near the benefit of passphrase + MFA
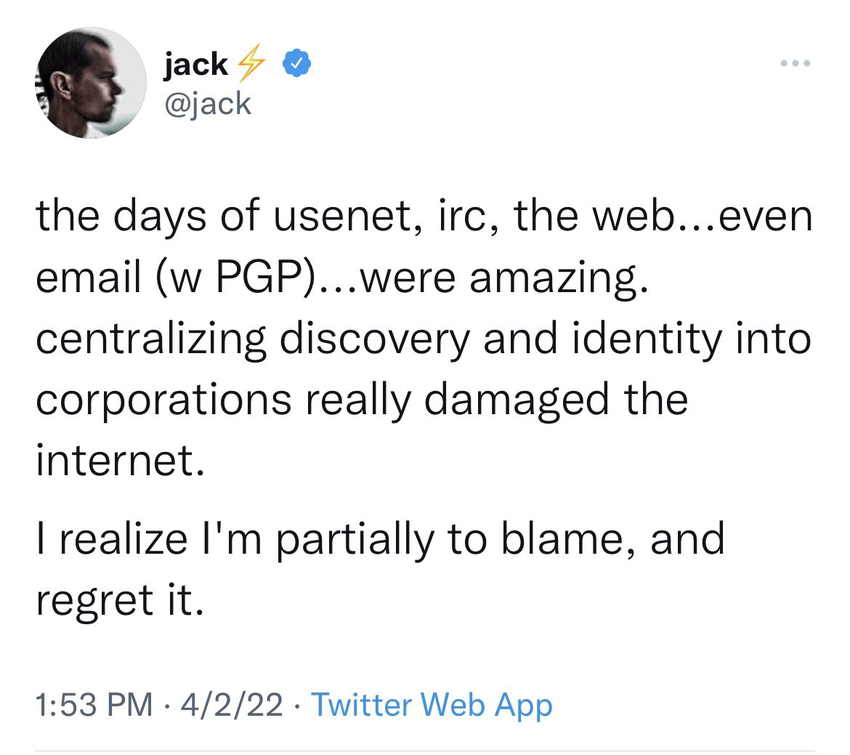
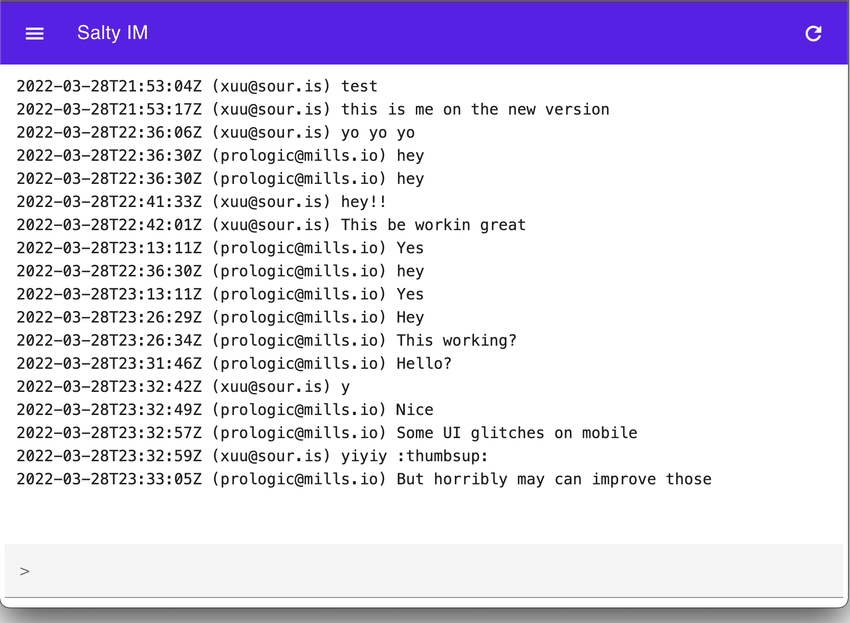
1.
#!/bin/sh
# Validate environment
if ! command -v msgbus > /dev/null; then
printf "missing msgbus command. Use: go install git.mills.io/prologic/msgbus/cmd/msgbus@latest"
exit 1
fi
if ! command -v salty > /dev/null; then
printf "missing salty command. Use: go install go.mills.io/salty/cmd/salty@latest"
exit 1
fi
if ! command -v salty-keygen > /dev/null; then
printf "missing salty-keygen command. Use: go install go.mills.io/salty/cmd/salty-keygen@latest"
exit 1
fi
if [ -z "$SALTY_IDENTITY" ]; then
export SALTY_IDENTITY="$HOME/.config/salty/$USER.key"
fi
get_user () {
user=$(grep user: "$SALTY_IDENTITY" | awk '{print $3}')
if [ -z "$user" ]; then
user="$USER"
fi
echo "$user"
}
stream () {
if [ -z "$SALTY_IDENTITY" ]; then
echo "SALTY_IDENTITY not set"
exit 2
fi
jq -r '.payload' | base64 -d | salty -i "$SALTY_IDENTITY" -d
}
lookup () {
if [ $# -lt 1 ]; then
printf "Usage: %s nick@domain\\n" "$(basename "$0")"
exit 1
fi
user="$1"
nick="$(echo "$user" | awk -F@ '{ print $1 }')"
domain="$(echo "$user" | awk -F@ '{ print $2 }')"
curl -qsSL "https://$domain/.well-known/salty/${nick}.json"
}
readmsgs () {
topic="$1"
if [ -z "$topic" ]; then
topic=$(get_user)
fi
export SALTY_IDENTITY="$HOME/.config/salty/$topic.key"
if [ ! -f "$SALTY_IDENTITY" ]; then
echo "identity file missing for user $topic" >&2
exit 1
fi
msgbus sub "$topic" "$0"
}
sendmsg () {
if [ $# -lt 2 ]; then
printf "Usage: %s nick@domain.tld <message>\\n" "$(basename "$0")"
exit 0
fi
if [ -z "$SALTY_IDENTITY" ]; then
echo "SALTY_IDENTITY not set"
exit 2
fi
user="$1"
message="$2"
salty_json="$(mktemp /tmp/salty.XXXXXX)"
lookup "$user" > "$salty_json"
endpoint="$(jq -r '.endpoint' < "$salty_json")"
topic="$(jq -r '.topic' < "$salty_json")"
key="$(jq -r '.key' < "$salty_json")"
rm "$salty_json"
message="[$(date +%FT%TZ)] <$(get_user)> $message"
echo "$message" \\
| salty -i "$SALTY_IDENTITY" -r "$key" \\
| msgbus -u "$endpoint" pub "$topic"
}
make_user () {
mkdir -p "$HOME/.config/salty"
if [ $# -lt 1 ]; then
user=$USER
else
user=$1
fi
identity_file="$HOME/.config/salty/$user.key"
if [ -f "$identity_file" ]; then
printf "user key exists!"
exit 1
fi
# Check for msgbus env.. probably can make it fallback to looking for a config file?
if [ -z "$MSGBUS_URI" ]; then
printf "missing MSGBUS_URI in environment"
exit 1
fi
salty-keygen -o "$identity_file"
echo "# user: $user" >> "$identity_file"
pubkey=$(grep key: "$identity_file" | awk '{print $4}')
cat <<- EOF
Create this file in your webserver well-known folder. https://hostname.tld/.well-known/salty/$user.json
{
"endpoint": "$MSGBUS_URI",
"topic": "$user",
"key": "$pubkey"
}
EOF
}
# check if streaming
if [ ! -t 1 ]; then
stream
exit 0
fi
# Show Help
if [ $# -lt 1 ]; then
printf "Commands: send read lookup"
exit 0
fi
CMD=$1
shift
case $CMD in
send)
sendmsg "$@"
;;
read)
readmsgs "$@"
;;
lookup)
lookup "$@"
;;
make-user)
make_user "$@"
;;
esac
#!/bin/sh
# Validate environment
if ! command -v msgbus > /dev/null; then
printf "missing msgbus command. Use: go install git.mills.io/prologic/msgbus/cmd/msgbus@latest"
exit 1
fi
if ! command -v salty > /dev/null; then
printf "missing salty command. Use: go install go.mills.io/salty/cmd/salty@latest"
exit 1
fi
if ! command -v salty-keygen > /dev/null; then
printf "missing salty-keygen command. Use: go install go.mills.io/salty/cmd/salty-keygen@latest"
exit 1
fi
if [ -z "$SALTY_IDENTITY" ]; then
export SALTY_IDENTITY="$HOME/.config/salty/$USER.key"
fi
get_user () {
user=$(grep user: "$SALTY_IDENTITY" | awk '{print $3}')
if [ -z "$user" ]; then
user="$USER"
fi
echo "$user"
}
stream () {
if [ -z "$SALTY_IDENTITY" ]; then
echo "SALTY_IDENTITY not set"
exit 2
fi
jq -r '.payload' | base64 -d | salty -i "$SALTY_IDENTITY" -d
}
lookup () {
if [ $# -lt 1 ]; then
printf "Usage: %s nick@domain\n" "$(basename "$0")"
exit 1
fi
user="$1"
nick="$(echo "$user" | awk -F@ '{ print $1 }')"
domain="$(echo "$user" | awk -F@ '{ print $2 }')"
curl -qsSL "https://$domain/.well-known/salty/${nick}.json"
}
readmsgs () {
topic="$1"
if [ -z "$topic" ]; then
topic=$(get_user)
fi
export SALTY_IDENTITY="$HOME/.config/salty/$topic.key"
if [ ! -f "$SALTY_IDENTITY" ]; then
echo "identity file missing for user $topic" >&2
exit 1
fi
msgbus sub "$topic" "$0"
}
sendmsg () {
if [ $# -lt 2 ]; then
printf "Usage: %s nick@domain.tld <message>\n" "$(basename "$0")"
exit 0
fi
if [ -z "$SALTY_IDENTITY" ]; then
echo "SALTY_IDENTITY not set"
exit 2
fi
user="$1"
message="$2"
salty_json="$(mktemp /tmp/salty.XXXXXX)"
lookup "$user" > "$salty_json"
endpoint="$(jq -r '.endpoint' < "$salty_json")"
topic="$(jq -r '.topic' < "$salty_json")"
key="$(jq -r '.key' < "$salty_json")"
rm "$salty_json"
message="[$(date +%FT%TZ)] <$(get_user)> $message"
echo "$message" \
| salty -i "$SALTY_IDENTITY" -r "$key" \
| msgbus -u "$endpoint" pub "$topic"
}
make_user () {
mkdir -p "$HOME/.config/salty"
if [ $# -lt 1 ]; then
user=$USER
else
user=$1
fi
identity_file="$HOME/.config/salty/$user.key"
if [ -f "$identity_file" ]; then
printf "user key exists!"
exit 1
fi
# Check for msgbus env.. probably can make it fallback to looking for a config file?
if [ -z "$MSGBUS_URI" ]; then
printf "missing MSGBUS_URI in environment"
exit 1
fi
salty-keygen -o "$identity_file"
echo "# user: $user" >> "$identity_file"
pubkey=$(grep key: "$identity_file" | awk '{print $4}')
cat <<- EOF
Create this file in your webserver well-known folder. https://hostname.tld/.well-known/salty/$user.json
{
"endpoint": "$MSGBUS_URI",
"topic": "$user",
"key": "$pubkey"
}
EOF
}
# check if streaming
if [ ! -t 1 ]; then
stream
exit 0
fi
# Show Help
if [ $# -lt 1 ]; then
printf "Commands: send read lookup"
exit 0
fi
CMD=$1
shift
case $CMD in
send)
sendmsg "$@"
;;
read)
readmsgs "$@"
;;
lookup)
lookup "$@"
;;
make-user)
make_user "$@"
;;
esac
make preflight
It just comes down to your threat model :)
A property of ec keys is deriving new keys that can be determined to be "on curve." bitcoin has some BIPs that derive single use keys for every transaction connected to a wallet. And be derived as either public or private chains. https://qvault.io/security/bip-32-watch-only-wallets/
> BREAKING: Russian billionaire Alisher Usmanov's super yacht, one of the biggest in the world, seized in Germany - Forbes
>
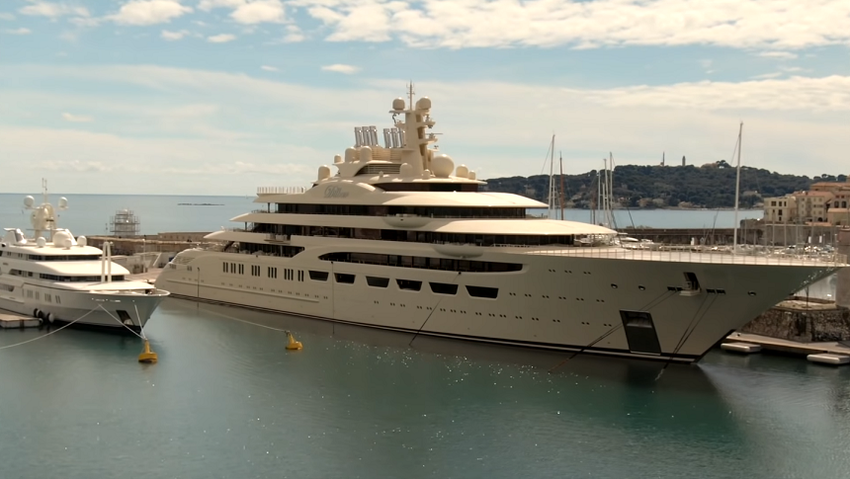
https://www.forbes.com/sites/giacomotognini/2022/03/02/germans-seize-russian-billionaire-alisher-usmanovs-mega-yacht/?sh=8b18f5052ddd
::1
⬛⬛⬛⬛⬛
🟩⬛⬛⬛⬛
🟩⬛🟨🟨⬛
🟩⬛⬛🟩🟩
🟩🟩🟩🟩🟩
⬛⬛⬛⬛⬛
🟩⬛⬛⬛⬛
🟩⬛🟨🟨⬛
🟩⬛⬛🟩🟩
🟩🟩🟩🟩🟩
⬛⬛⬛⬛⬛
⬛⬛🟨🟨⬛
⬛🟨🟩⬛🟨
🟩🟩🟩🟩🟩
⬛⬛⬛⬛⬛
⬛⬛🟨🟨⬛
⬛🟨🟩⬛🟨
🟩🟩🟩🟩🟩
⬛⬛🟨⬛⬛
🟩⬛⬛🟩🟩
🟩🟩🟩🟩🟩
⬛⬛🟨⬛⬛
🟩⬛⬛🟩🟩
🟩🟩🟩🟩🟩
Try https://twtxt.net!
⬛🟨🟨⬛⬛
🟨🟨⬛⬛⬛
⬛⬛🟨🟨🟩
🟩🟩🟩🟩🟩
⬛🟨🟨⬛⬛
🟨🟨⬛⬛⬛
⬛⬛🟨🟨🟩
🟩🟩🟩🟩🟩
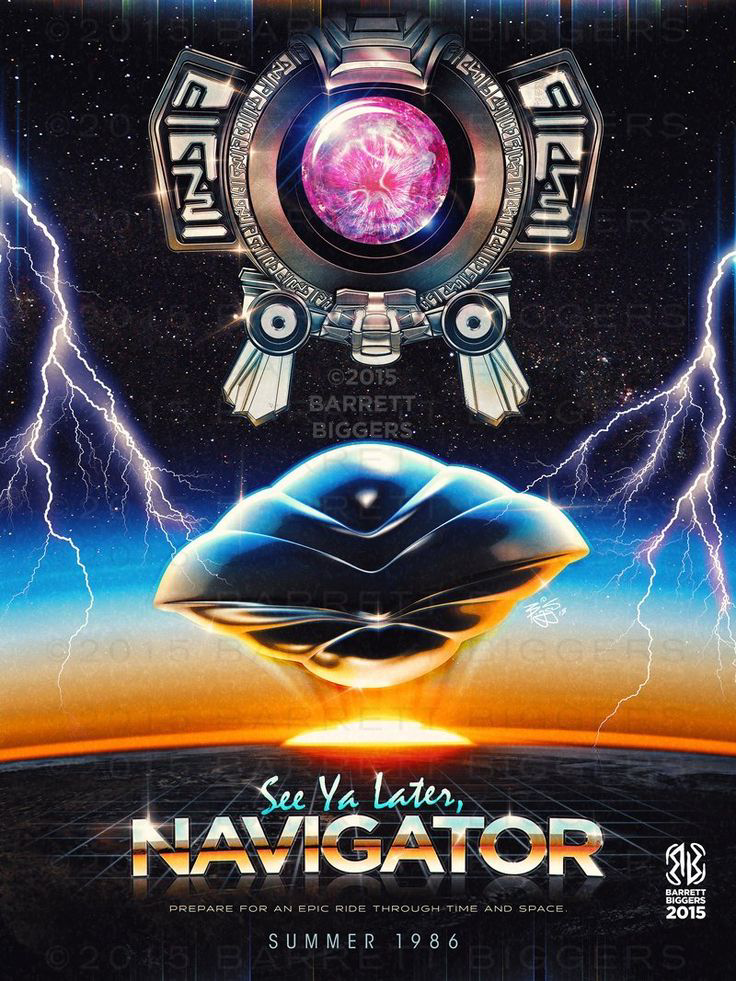
🟨🟨⬛🟨🟨
🟨🟨🟩⬛🟨
🟩🟩🟩🟩🟩
🟨🟨⬛🟨🟨
🟨🟨🟩⬛🟨
🟩🟩🟩🟩🟩
Wordle 220 4/6*
⬛🟨🟨⬛⬛
⬛🟨🟨⬛⬛
⬛🟩⬛🟩🟩
🟩🟩🟩🟩🟩
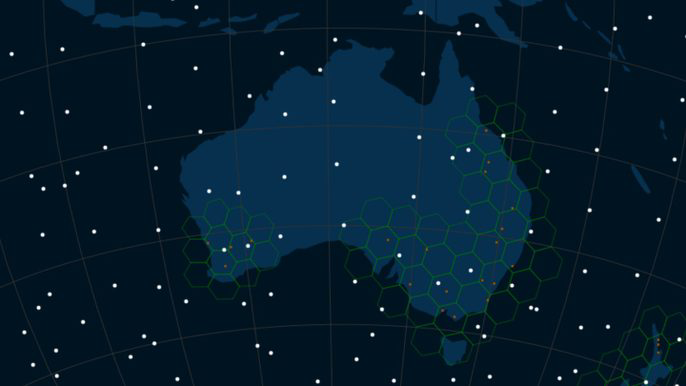
Do they have Starlink beta down there yet?
Wordle 219 4/6*
⬛⬛⬛⬛⬛
🟨🟨⬛⬛⬛
⬛🟨🟩⬛⬛
🟩🟩🟩🟩🟩
Wordle 217 4/6
⬛🟩🟩⬛⬛
⬛🟩🟩🟨⬛
⬛🟩🟩⬛🟩
🟩🟩🟩🟩🟩
⬛⬛⬛⬛🟨
🟩🟩⬛⬛⬛
🟩🟩🟩🟩⬛
🟩🟩🟩🟩🟩
⬛⬛⬛⬛🟨
⬛⬛⬛⬛⬛
🟩⬛🟨⬛🟨
🟩🟩🟩🟩🟩
On SmartTV however this would be a nice addition.
On SmartTV however this would be a nice addition.
I remember similar things back in dialup days where your ISP would proxy things to you and supercompress the images.
A render mode for Gemini probably wouldnt be too hard. There are markdown to Gemini libs out there.
With Web3 the whole trust a 3rd party browser ext + high fees + env impact for compute and storage are serious no gos for me.. I have heard one too many horror stories about clicking the wrong link and some script draining your metamask wallet.
vs.
I liked words where X make a Z sound. And also had a bit of dyslexia so my firs IRC nick was Xypher swapping the y and e.. I would also use the forms Xypherius or just Xypheri.
Because its close hemming to Cypher I found the nick would get used by others.. Though that is not my origin.
Later I would sign websites I created as The X-Urban Underground (where X was short for Xypher) and that evolved to xuu. Pronounced like zoo.